About
ILINX eForms, a key component of ILINX Capture and the ILINX ECM Platform, enables you to easily capture accurate information from a desktop, laptop, tablet or smartphone. Where the out-of-the-box functionality is mostly point-and-click and comes with a number of versatile features, ILINX eForms also allows you to customize the interface and processes.
This technical guide contains information on how to configure the ILINX eForms Administrator tools and options that for developers to create custom business processes and interfaces. There are core tools that can be used in the development of these process using jQuery.
Note: This guide assumes that installation of the ILINX Capture Platform and ILINX Web Platform have taken place. For complete installation documentation, please see the ILINX Installation Guide
Note: ImageSource provides limited support for load-balancing ILINX eForms. If ILINX Web Platform has been installed on multiple server instances, ensure that “sticky sessions” are enabled. After creating a custom theme or making edits to a custom theme, the content of the “ILINX Web Platform\eFormsApp\data\themes” folder should be copied from the server which has those updated themes to the same folder on all other server instances
Introduction
This guide provides basic information for applying scripts to ILINX eForms. While ILINX eForms has a GUI front end using drag n drop fields to the form, you can use scripts to apply additional validation, element design, and dynamic changes. Scripts can be used through the ILINX Capture workflow processes as well as using CSS for any design template requirements. Dynamic webpage changes within ILINX eForms are done using jQuery script to modify values as well as CSS.
What are dynamic eForms?
The term “dynamic” refers to the use of JavaScript to be able to do customizations of eForms including changing the look after data is entered and use of calculations.
> Example of manipulating value:
– Calculating total cost
– Express as decimal values
– Calculating a date
> Example of design elements you can change are:
– the color of a textbox
– alignment of field values
– set readOnly property
Initial Configuration
Minimal Script Required
JavaScript is used as a coding standard to create dynamic changes on the form. The example code below does not provide functionality by itself. It is the basic requirement for jQuery as well as usability for ILINX eForms. You will notice all the code you type will require these basic scripts and functions.
$(function () { // Change the property below and add your own custom code. EFormScripting.enabledOnBatchCreate = false; EFormScripting.enabledOnBatchOpen = false; EFormScripting.enabledOnBatchClosing = false; EFormScripting.enabledOnFieldEnter = false; EFormScripting.enabledOnFieldExit = false; EFormScripting.onBatchCreate = function() { // Custom code } EFormScripting.onBatchOpen = function() { // Custom code } EFormScripting.onBatchClosing = function() { // Custom code // Returns true to submit this form and false to not submit return true; } EFormScripting.onFieldEnter = function(field_id, field_value){ // Custom code } EFormScripting.onFieldExit = function(field_id, field_value){ // Custom code } // Register custom script. Do not remove. EFormScripting.registeredCustomEvent(); })
Examples
Sample code is provided as part of the ILINX Capture Platform installation. Navigating to [ILINX Capture installation folder]\Samples Code\EFormClientScript Sample\ includes a JScript Script file that can be edited as desired. These custom methods can be enabled and modified:
onBatchCreate
EFormScripting.onBatchCreate = function() { DebugToConsole("EFormScripting.onBatchCreate() Enter"); try { SetTheFormOnBatchCreation(); }catch (err) { LogErrorToConsole(err); } DebugToConsole("EFormScripting.onBatchCreate() Exit"); }
onBatchOpen
EFormScripting.onBatchOpen = function() { DebugToConsole("EFormScripting.onBatchOpen() Enter"); try { SignStamp(); // hides the hidden textbox based on if checkbox is checked or not // Get the current queue to enable the elements for that queue switch (document.getElementById("element_39").value) { //Current Queue case "Employee Queue": SetFormForEmployeeQueue(); break; case "HR Queue": SetFormForHrQueue(); break; case "Manager Queue": SetFormForManagersQueue(); break; case "Payroll Queue": //DisabledAllFieldsOnForm(); break; case "Support Error Queue": //DisabledAllFieldsOnForm(); break; default: //DisabledAllFieldsOnForm(); break; } }catch (err) { LogErrorToConsole(err); } DebugToConsole("EFormScripting.onBatchOpen() Exit"); }
onBatchClosing
EFormScripting.onBatchClosing = function() { DebugToConsole("EFormScripting.onBatchClosing() Enter"); var retBool = false; try { // Enable the eForm fields during submit so the values get committed to the database document.getElementById("element_4").removeAttribute("disabled"); // Manager document.getElementById("element_40").removeAttribute("disabled"); // Decision document.getElementById("element_9_1").removeAttribute("disabled"); // Leaving The US document.getElementById("element_2").removeAttribute("disabled"); // Start Date document.getElementById("element_3").removeAttribute("disabled"); // End Date document.getElementById("UserMessage").innerHTML = ""; // Custom Element Error Message if (DataIsValid()) { retBool = true; } }catch (err) { LogErrorToConsole(err); retBool = false; } DebugToConsole("EFormScripting.onBatchClosing() Exit"); return retBool; }
onFieldEnter
EFormScripting.onFieldEnter = function(field_id, field_value){ // The following controls are capable of receiving onFieldEnter and/or onFieldExit event: // // - Single Line Text // - Number // - Text Area // - Checkboxes // - Radio buttons // - Drop Down // - Date // - Time // - Table fields // - Matrix Choice // - Amount // - Website // - Name // - Address // - Email // - Phone alert("onFieldEnter: " + field_id); }
onFieldExit
EFormScripting.onFieldExit = function(field_id, field_value){ // The following controls are capable of receiving onFieldEnter and/or onFieldExit event: // // - Single Line Text // - Number // - Text Area // - Checkboxes // - Radio buttons // - Drop Down // - Date // - Time // - Table fields // - Matrix Choice // - Amount // - Website // - Name // - Address // - Email // - Phone // Decide which control ID is receiving the focus if (field_id == "element_1" && field_value == "focused") { // Set the focus back to the control $("#" + field_id).focus(); }else { alert("onFieldExit: " + field_id); } }
Display Elements
When each field is created, it is assigned an Element ID in the database. This ID is used as the control when you want to display or modify a value, updating or changing the field look, make modifications to the field properties, etc. The Element ID may also be referred to as the Control ID when viewing in the eForms UI or Field ID when developing in a script.
To identify the element ID for any field:
Step 1: Add a field to your form.
Step 2: Select the field, click the Field Properties tab.
Step 3: Towards the end of the properties panel the Control ID will be displayed.
This is the element ID to use within your custom scripts.
Deploying Script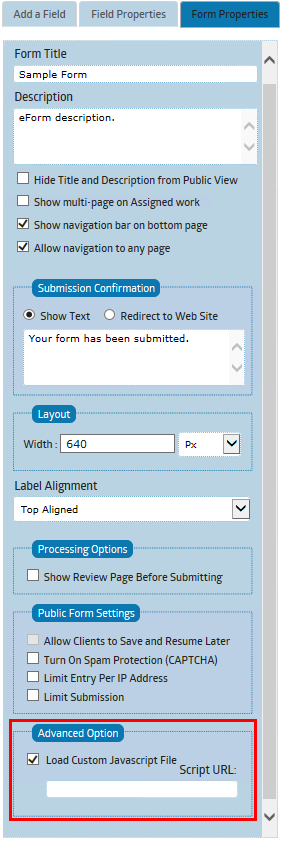
Developing the script may be done on any preferred platform. Some options include Microsoft Visual Studios or Notepad++. Once your script is developed within your preferred platform, the file must be saved as a script file using a .js extension (i.e. examplescript.js).
Upload the script file to valid URL (i.e. http://www.example.com/examplescript.js).
To attach your custom script:
Step 1: Open your form.
Step 2: Select the Form Properties tab.
Step 3: Towards the end of the properties panel, under Advanced Option, enable the Load Custom Javascript File checkbox.
Step 4: Add the URL location where you uploaded the .js custom script to the Script URL box.
Note: Only one script may be loaded for each form.
Examples
Field Values
To get the value of a given field, you can use the getElementbyID Method.
Price= document.getElementById("element_38").value;
Modifying Values
The example below is multiplying two elements; “element_1” = Price & “element_2” = Quantity. The “element_3” field will be modified with the Total Value.
$(function () { EFormScripting.enabledOnFieldExit = true; EFormScripting.onFieldExit = function (field_id, field_value) { if (field_id == "element_1" || field_id == "element_2") { var price = document.getElementById("element_1").value; var quantity = document.getElementById("element_2").value; var total = 0; //alert(field_id); if (price == null && quantity == null) { total = null; document.getElementById("element_3").value = total; } else { total = price * quantity; document.getElementById("element_3").value = total; } } } // Register custom script. Do not remove. EFormScripting.registeredCustomEvent(); })
Decimal Values
It may be necessary to display a value in a decimal format using a fixed length.
Syntax to change a price field into a two decimal fixed length:
price.value = parseFloat(price.value).toFixed(2);
To calculate the Total field value into two decimal fixed lengths:
total.value = (price.value * quantity.value).toFixed(2);
The example below is multiplying two elements; “element_1” = Price & “element_2” = Quantity. The “element_3” field will be modified with the Total Value to the fixed two decimal length.
$(function () { // Creating Alias for Field id var price = document.getElementById("element_1"); var quantity = document.getElementById("element_2"); var total = document.getElementById("element_3"); EFormScripting.enabledOnFieldExit = true; EFormScripting.onFieldExit = function (field_id, field_value) { if (field_id == price.id || field_id == quantity.id) { price.value = parseFloat(price.value).toFixed(2); // alert(field_id); if (price.value == null && quantity.value == null) { total.value = null; } else { total.value = (price.value * quantity.value).toFixed(2); } } } // Register custom script. Do not remove. EFormScripting.registeredCustomEvent(); })
ReadOnly
Some fields may need to be set to Read Only to ensure users are not able to make changes.
$(function () { // Creating Alias for Field id var price = document.getElementById("element_1"); var quantity = document.getElementById("element_2"); var total = document.getElementById("element_3"); total.readOnly = true; EFormScripting.enabledOnFieldExit = true; EFormScripting.onFieldExit = function (field_id, field_value) { if (field_id == price.id || field_id == quantity.id) { price.value = parseFloat(price.value).toFixed(2); // alert(field_id); if (price.value == null && quantity.value == null) { total.value = null; } else { total.value = (price.value * quantity.value).toFixed(2); } } } // Register custom script. Do not remove. EFormScripting.registeredCustomEvent(); })
Conditional Requirements
At times it is necessary to set conditions on field elements. The below syntax sets “element_2” required ONLY when “element_1” equals “test”. This required value should be set outside of the onFieldExit or the condition could be bypassed just by changing the values.
$(function () { // Creating Alias for Field id var firsttextbox = document.getElementById("element_1"); var secondtextbox = document.getElementById("element_2"); EFormScripting.enabledOnFieldExit = true; secondtextbox.required = true; EFormScripting.onFieldExit = function (field_id, field_value) { if (field_id == firsttextbox.id && field_value != "test") { // alert(field_id); secondtextbox.required = false; } } // Register custom script. Do not remove. EFormScripting.registeredCustomEvent(); })
Multiple Inputs
When an eForm field includes multiple inputs (ie. the Amount field), it may be necessary to modify each input separately. Each input may be referenced such as:
> Control ID = “element_5”
– Dollars input = “element_5_1”
– Cents input = “element_5_2”
Modifying CSS
The CSS can also be modified using scripting. The example below will:
> Right align all text
> Set the field background color to pink if the total value is < 0
> Otherwise set the field background color to green
$(function () { //using alias var total = document.getElementById("element_1"); //enable On Field Exit EFormScripting.enabledOnFieldExit = true; //On field exit function EFormScripting.onFieldExit = function (field_id, field_value) { if ((field_id == total.id) && (field_value < 0)) { $("#" + total.id).css({ "text-align": "right", "background-color": "pink" }); } else { $("#" + total.id).css({ "text-align": "right", "background-color": "lime" }); } } // Register custom script. Do not remove. EFormScripting.registeredCustomEvent(); })
For more information refer to Jquery using the CSS method.
Dates
Today
The examples below sets the date field value to Today’s date.
$(function () { var DateField = document.getElementById("element_1"); var today = new Date(); var dd = today.getDate(); var mm = today.getMonth() + 1; var yyyy = today.getFullYear(); if (dd < 10) { dd = '0' + dd; } if (mm < 10) { mm = '0' + mm; } DateField.value = mm + '/' + dd + '/' + yyyy; //enable On Field Exit EFormScripting.enabledOnFieldExit = true; //On field exit function EFormScripting.onFieldExit = function (field_id, field_value) { //alert("onFieldExit: " + field_id); } // Register custom script. Do not remove. EFormScripting.registeredCustomEvent(); })
Adding 60 days
The syntax below provides an example of setting the field value 60 days in the future of today’s date.
$(function () { Date.prototype.addDays = function (days) { var date = new Date(this.valueOf()); date.setDate(date.getDate() + days); return date; } var DateField = document.getElementById("element_1"); today = new Date(); date = today.addDays(60); var dd = date.getDate(); var mm = date.getMonth() + 1; var yyyy = date.getFullYear(); if (dd < 10) { dd = '0' + dd; } if (mm < 10) { mm = '0' + mm; } DateField.value = mm + '/' + dd + '/' + yyyy; //enable On Field Exit EFormScripting.enabledOnFieldExit = true; //On field exit function EFormScripting.onFieldExit = function (field_id, field_value) { //alert("onFieldExit: " + field_id); } // Register custom script. Do not remove. EFormScripting.registeredCustomEvent(); })
Sending a Message
Displaying messages to the user may be necessary. The syntax below is an example to display “Text to the end user.”
$(function () { function ShowMessageToUser(messageToUser) { document.getElementById("UserMessage").innerHTML = messageToUser; } var userMessageTag = document.createElement('h3'); userMessageTag.style.color = "red"; userMessageTag.id = "UserMessage" document.getElementById("main_body").appendChild(userMessageTag); ShowMessageToUser("Text to the end user"); // Register custom script. Do not remove. EFormScripting.registeredCustomEvent(); })
Tables
Tables may be created using an array. In the example below, a SubTotal and Total row is scripted with a fixed decimal.
$(function () { //enable On Field Exit EFormScripting.enabledOnFieldExit = true; //On field exit function EFormScripting.onFieldExit = function (field_id, field_value) { var myTable = document.getElementById("element_1"); var rowCount = myTable.rows.length; console.log("rowlength: " + myTable.rows.length); debug = true var i = 0 var sum = 0.0; for (var i = 0; i < rowCount; ++i) { PriceID = "element_1_" + i + "_0" if (debug == true) { console.log("PriceID: " + PriceID); } try { Price = document.getElementById(PriceID).value; } catch (e) { Price=null } if (Price) { document.getElementById(PriceID).value = (parseFloat(Price)).toFixed(2); } //You need to prefix price with a plus sign, so it does not get interpreted as a string sum = sum + +Price if (debug == true) { console.log("SubTotal: " + (parseFloat(sum)).toFixed(2)); } } if (debug == true) { console.log("Total: " + (parseFloat(sum)).toFixed(2)); } } // Register custom script. Do not remove. EFormScripting.registeredCustomEvent(); })
SSO Integration
A sample web application that demonstrates how to integrate eForms using SSO is available in the sample project inside the ILINXCaptureSample.zip file. Refer to the EFormSSO sample project where appropriate code comments and examples are provided.
Troubleshooting
There are a few basic things to verify if you are having issues validating your script.
> Verify the Field_Id (element_id) to ensure it matches the ID on your form.
> Verify each statement is terminated with a semicolon (;).
> Add an alert function within the script to make sure it is running.
> If the script is not running, check the website location the script was uploaded. Verify the URL is correct within the configured eForm.
Write to Console Log
To ensure the correct values are written or configured, you can write the values to the console. Example syntax:
$(function () { console.log("Script Started"); // Register custom script. Do not remove. EFormScripting.registeredCustomEvent(); })